CAPTCHAFORUM
Administrator
Text Captcha is a type of captcha that is represented as text and doesn't contain images. Usually you have to answer a question to pass the verification.
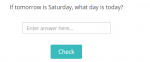
1.Find captcha question.
2.Send question to 2Captcha API.
PHP
PYTHON
JAVA
CSHARP
GO
CPP
3. Paste received code into the field. Then, submit the form.
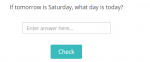
1.Find captcha question.
2.Send question to 2Captcha API.
PHP
PHP:
// https://github.com/2captcha/2captcha-php
require(__DIR__ . '/../src/autoloader.php');
$solver = new \TwoCaptcha\TwoCaptcha('YOUR_API_KEY');
try {
$result = $solver->text([
'text' => 'If tomorrow is Saturday, what day is today?',
'lang' => 'en',
]);
} catch (\Exception $e) {
die($e->getMessage());
}
die('Captcha solved: ' . $result->code);
PYTHON
Python:
# https://github.com/2captcha/2captcha-python
import sys
import os
sys.path.append(os.path.dirname(os.path.dirname(os.path.realpath(__file__))))
from twocaptcha import TwoCaptcha
api_key = os.getenv('APIKEY_2CAPTCHA', 'YOUR_API_KEY')
solver = TwoCaptcha(api_key, defaultTimeout=40, pollingInterval=10)
try:
result = solver.text('If tomorrow is Saturday, what day is today?',
lang='en')
except Exception as e:
sys.exit(e)
else:
sys.exit('solved: ' + str(result))
JAVA
Java:
// https://github.com/2captcha/2captcha-java
package examples;
import com.twocaptcha.TwoCaptcha;
import com.twocaptcha.captcha.Text;
public class TextExample {
public static void main(String[] args) {
TwoCaptcha solver = new TwoCaptcha("YOUR_API_KEY");
Text captcha = new Text();
captcha.setText("If tomorrow is Saturday, what day is today?");
captcha.setLang("en");
try {
solver.solve(captcha);
System.out.println("Captcha solved: " + captcha.getCode());
} catch (Exception e) {
System.out.println("Error occurred: " + e.getMessage());
}
}
}
CSHARP
Code:
// https://github.com/2captcha/2captcha-csharp
using System;
using System.Linq;
using TwoCaptcha.Captcha;
namespace TwoCaptcha.Examples
{
public class TextExample
{
public void Main()
{
TwoCaptcha solver = new TwoCaptcha("YOUR_API_KEY");
Text captcha = new Text();
captcha.SetText("If tomorrow is Saturday, what day is today?");
captcha.SetLang("en");
try
{
solver.Solve(captcha).Wait();
Console.WriteLine("Captcha solved: " + captcha.Code);
}
catch (AggregateException e)
{
Console.WriteLine("Error occurred: " + e.InnerExceptions.First().Message);
}
}
}
}
GO
Code:
// https://github.com/2captcha/2captcha-go
package main
import (
"fmt"
"log"
"github.com/2captcha/2captcha-go"
)
func main() {
client := api2captcha.NewClient("API_KEY")
cap := api2captcha.Text{
Text: "If tomorrow is Saturday, what day is today?",
Lang: "en",
}
code, err := client.Solve(cap.ToRequest())
if err != nil {
log.Fatal(err);
}
fmt.Println("code "+code)
}
CPP
Code:
// https://github.com/2captcha/2captcha-cpp
#include <cstdio>
#include "curl_http.hpp"
#include "api2captcha.hpp"
int main (int ac, char ** av)
{
if (ac < 2)
{
printf ("Usage: ./text \"Your question\"\n");
return 0;
}
api2captcha::curl_http_t http;
http.set_verbose (true);
api2captcha::client_t client;
client.set_http_client (&http);
client.set_api_key (API_KEY);
assert (ac > 1);
api2captcha::text_t cap (av[1]);
try
{
client.solve (cap);
printf ("code '%s'\n", cap.code ().c_str ());
}
catch (std::exception & e)
{
fprintf (stderr, "Failed: %s\n", e.what ());
}
return 0;
}
3. Paste received code into the field. Then, submit the form.